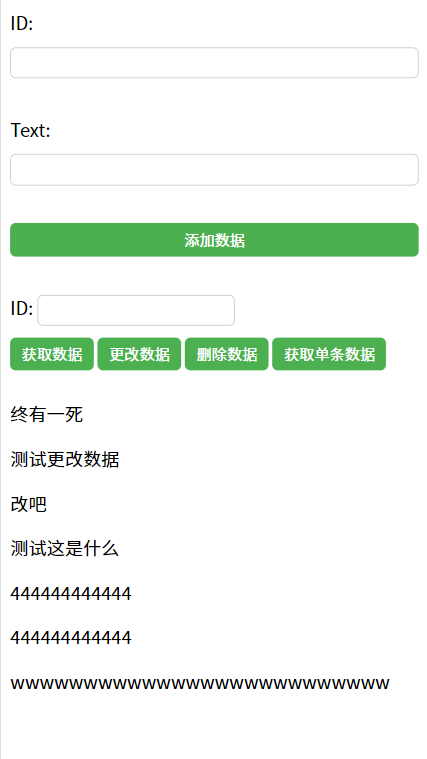
前后端文件NodeMongoose (2).zip
前端代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fetch 学习</title>
<style>
form{
display:flex;
flex-direction:column;
}
label{
margin-bottom:10px;
}
input{
padding:5px;
margin-bottom:10px;
border-radius:5px;
border:1px solid #ccc;
}
button{
padding:5px 10px;
margin-bottom:10px;
border-radius:5px;
border:none;
background-color:#4CAF50;
color:#fff;
cursor:pointer;
}
</style>
</head>
<body>
<form action="#" class="add">
<label for="id">ID:</label>
<input type="number" id="id" name="id" required>
</br>
<label for="text">Text:</label>
<input type="text" id="text" name="text" required>
</br>
<button type="submit">添加数据</button>
</form>
</br>
<label for="getId">ID:</label>
<input type="number" id="getId" name="getId" required>
</br>
<button id="getBtn">获取数据</button>
<button id="updateBtn">更改数据</button>
<button id="deleteBtn">删除数据</button>
<button id="getOneBtn">获取单条数据</button>
<p id="result"></p>
</body>
<script>
//get请求
async function getQuotes(){
const res = await fetch('http://localhost:3000/quotes')
const data = await res.json()
console.log(data)
document.getElementById('result').innerHTML = data.data.map(item => `<p>${item.text}</p>`).join('')}
//post请求
async function addQuote(id,text){
let data = {
id,
text
}
const res = await fetch('http://localhost:3000/add',{
method:'POST',
headers:{
'Content-Type':'application/json'
},
body:JSON.stringify(data)
})
const result = await res.json()
console.log(result)
}
//put请求
async function updateQuote(id,text){
let data = {
id,
text
}
const res = await fetch('http://localhost:3000/update',{
method:'PUT',
headers:{
'Content-Type':'application/json'
},
body:JSON.stringify(data)
})
const result = await res.json()
console.log(result)
}
//delete请求
async function deleteOne(id){
const res = await fetch(`http://localhost:3000/delete/${id}`,{
method:'DELETE',
headers:{
'Content-Type':'application/json'
},
data:JSON.stringify(id)
})
const result = await res.json()
console.log(result)
}
//获取单条数据
async function getQuotesOne(id){
const res = await fetch(`http://localhost:3000/quotes?id=${id}`)
const data = await res.json()
console.log(data)
document.getElementById('result').innerHTML = `<p>${data.data.text}</p>`
}
getQuotes()
</script>
<script>
document.getElementById('getBtn').addEventListener('click',async()=>{
await getQuotes()
})
document.getElementById('updateBtn').addEventListener('click',async()=>{
await updateQuote(4,'测试更改数据了')
getQuotes()
})
document.getElementById('deleteBtn').addEventListener('click',async()=>{
const id = document.getElementById('getId').value
await deleteOne(id)
getQuotes()
})
document.querySelector('.add').addEventListener('submit',async(e)=>{
e.preventDefault()
const id = e.target.elements.id.value
const text = e.target.elements.text.value
await addQuote(id,text)
e.target.reset()
getQuotes()
})
document.getElementById('getOneBtn').addEventListener('click',async()=>{
const id = document.getElementById('getId').value
await getQuotesOne(id)
})
</script>
</html>